Access Modifiers in C# with Program Examples
Access Modifiers in C#: An Overview
Understanding access specifiers in C# is fundamental to controlling the visibility and accessibility of classes, methods, and variables within your codebase. This article explores the nuances of public, private, protected, and internal access modifiers, guiding developers on how to design secure and efficient C# applications.
What are Access Modifiers in C#?
Access modifiers in C# control the visibility and accessibility of classes, methods, and variables. They define who can access and interact with these members, providing security and encapsulation in object-oriented programming, with options like public, private, protected, internal, and more.
Types of Access Modifiers in C#
In C#, there are 6 different types of Access Modifiers:
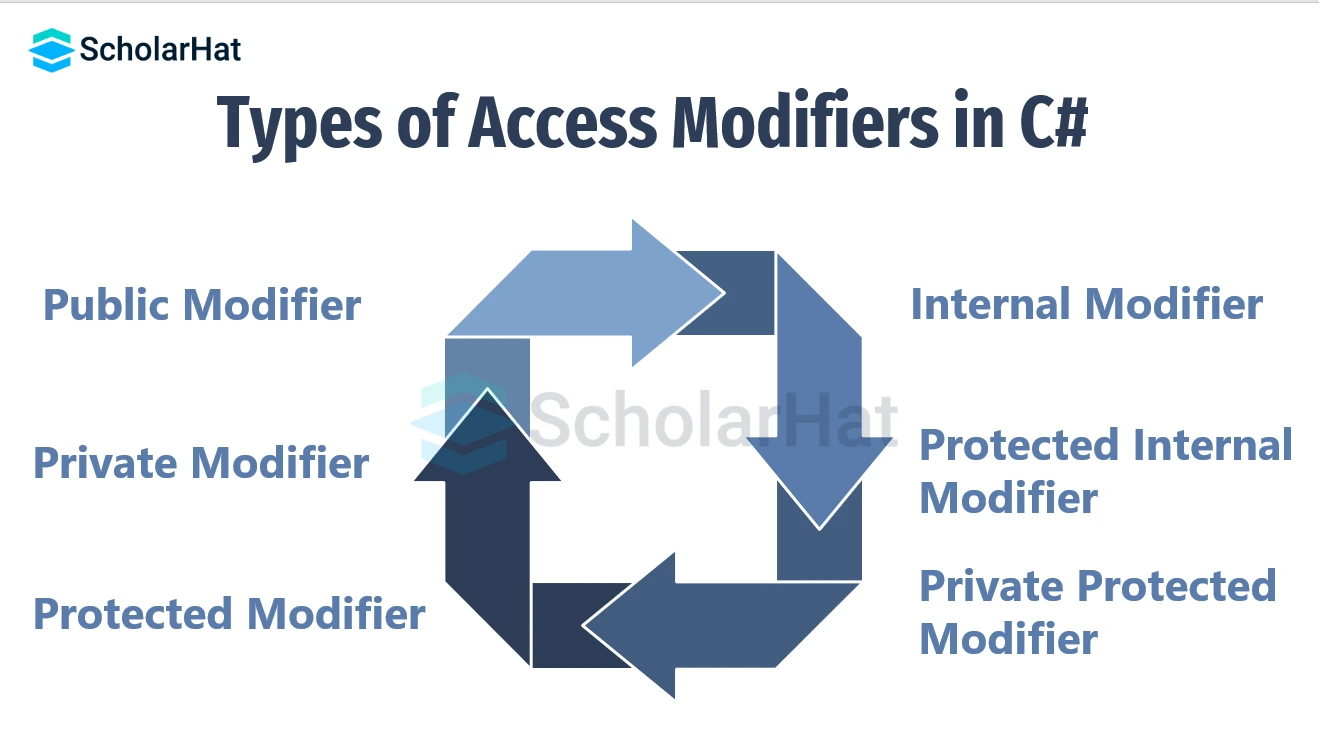
public | protected | internal | protected internal | private | private protected | |
Entire program | Yes | No | No | No | No | No |
Containing class | Yes | Yes | Yes | Yes | Yes | Yes |
Current assembly | Yes | No | Yes | Yes | No | No |
Derived types | Yes | Yes | No | Yes | No | No |
Derived types within current assembly | Yes | Yes | Yes | Yes | No | Yes |
Read More - C Sharp Interview Questions
1. Public Access Modifier in C#
In C#, the 'public' access modifier allows classes, methods, and variables to be accessed from any part of the program, making them widely accessible. It promotes encapsulation and facilitates interaction between different components, enabling seamless communication in object-oriented programming.
Example
using System;
namespace MyApplication
{
class Student
{
public string name = "Urmi";
public void print()
{
Console.WriteLine("Hello from Student class");
}
}
class Program
{
static void Main(string[] args)
{
// creating an object of the Student class
Student student1 = new Student();
// accessing the name field and printing it
Console.WriteLine("Name: " + student1.name);
// accessing the print method from the Student class
student1.print();
}
}
}
Explanation
This C# code in the C# Compiler defines a Student class with a name field and a print method. It creates an object, accesses fields/methods, and prints output.
Output
Name: Urmi
Hello from Student class
2. Protected Access Modifier in C#
In C#, the protected access modifier restricts member accessibility within the defining class and its derived classes. It enables inheritance, allowing subclasses to access protected members, ensuring data encapsulation and secure extension of base class functionality in object-oriented programming.
Syntax
protected TypeName
Example
using System;
namespace MyApplication
{
class Student
{
protected string name = "Urmi";
}
class Program
{
static void Main(string[] args)
{
// creating an object of the Student class
Student student1 = new Student();
// accessing the name field and printing it
Console.WriteLine("Name: " + student1.name);
}
}
}
Explanation
This C# code defines a Student class with a protected name field, accesses and prints the field in Main.
Output
Error CS0122 'Student.name' is inaccessible due to its protection level
3. Internal Access Modifier in C#
In C#, the internal access modifier restricts the access of a member within its own assembly. Members marked as internal can be accessed by other classes within the same assembly, providing encapsulation and ensuring that the implementation details are hidden from external assemblies.
Syntax
internal TypeName
Example
// C# Program to show use of internal access modifier inside the file Program.cs
using System;
namespace internalAccessModifier {
// Declare class Complex as internal
internal class Complex {
int real;
int img;
public void setData(int r, int i)
{
real = r;
img = i;
}
public void displayData()
{
Console.WriteLine("Real = {0}", real);
Console.WriteLine("Imaginary = {0}", img);
}
}
// Driver Class
class Program {
// Main Method
static void Main(string[] args)
{
// Instantiate the class Complex
// in separate class but within
// the same assembly
Complex c = new Complex();
// Accessible in class Program
c.setData(3, 2);
c.displayData();
}
}
}
Explanation
This C# code in the C# Compiler demonstrates the use of the internal access modifier. It defines an internal class Complex with private fields and public methods. The Program class, within the same assembly, creates an instance of Complex, sets data, and displays it, showcasing internal access within the assembly.
Output
Real = 3
Imaginary = 2
4. Protected Internal Access Modifier in C#
The protected internal access modifier in C# allows member access within the current assembly (internal) and by derived classes (protected). It combines the features of both modifiers, enabling restricted access within the assembly and to inheriting classes, promoting flexibility in class design while maintaining control over accessibility.
Syntax
protected internal TypeName
Example
// Inside file parent.cs
using System;
public class Parent
{
// Declaring member as protected internal
protected internal int value;
}
class ABC
{
// Trying to access
// value in another class
public void testAccess()
{
// Member value is Accessible
Parent obj1 = new Parent();
obj1.value = 12;
}
}
namespace DNT
{
class Child : Parent
{
// Main Method
public static void Main(String[] args)
{
// Accessing value in another assembly
Child obj3 = new Child();
// Member value is Accessible
obj3.value = 16;
Console.WriteLine("Value = " + obj3.value);
}
}
}
Explanation
In C#, Child class in namespace DNT accesses and modifies a member value from Parent class in another assembly.
Output
Value = 16
5. Private Access Modifier in C#
When we declare a type member with the private access modifier, it can only be accessed within the same class or structure.Example
using System;
namespace MyApplication {
class ScholarHat {
private string name = "ScholarHat";
private void print() {
Console.WriteLine("Welcome to ScholarHat class");
}
}
class Program {
static void Main(string[] args) {
// creating object of ScholarHat class
ScholarHat obj = new ScholarHat();
// accessing name field and printing it
Console.WriteLine("Name: " + obj.name);
// accessing print method from ScholarHat
obj.print();
Console.ReadLine();
}
}
}
Explanation
Since the field and method are private in the ScholarHat class, we are not able to access them from the Program class. Here, the code will generate the following error.Output
/tmp/fhz9gliRNs.cs(20,40): error CS0122: 'ScholarHat.name' is inaccessible due to its protection level
/tmp/fhz9gliRNs.cs(23,11): error CS0122: 'ScholarHat.print()' is inaccessible due to its protection level
/tmp/fhz9gliRNs.cs(6,20): warning CS0414: The field 'ScholarHat.name' is assigned but its value is never used
6. Private Protected Access Modifier in C#
The "private protected" access modifier in C# restricts access to members within the same class or derived classes from the same assembly, striking a balance between privacy and limited accessibility, ensuring that only closely related classes can access the member.
Syntax
private protected TypeName
Example
// C# Program to show use of// the private protected
// Accessibility Level
using System;
namespace PrivateProtectedAccessModifier {
class Parent {
// Member is declared as private protected
private protected int value;
// value is Accessible only inside the class
public void setValue(int v)
{
value = v;
}
public int getValue()
{
return value;
}
}
class Child : Parent {
public void showValue()
{
// Trying to access value
// Inside a derived class
Console.WriteLine("Value = " + value);
// value is accessible
}
}
// Driver Code
class Program {
// Main Method
static void Main(string[] args)
{
Parent obj = new Parent();
// obj.value = 5;
// Also gives an error
// Use public functions to assign
// and use value of the member 'value'
obj.setValue(4);
Console.WriteLine("Value = " + obj.getValue());
}
}
}
Explanation
This C# code in the C# Editor demonstrates the use of the private protected access modifier. It defines a Parent class with a private protected member 'value'. The Child class, inheriting from Parent, accesses and displays the 'value'. The Main method showcases how to set and retrieve 'value' using public functions due to its restricted access.
Output
Value = 4
Important Points to Remember of Access Modifiers in C#
- Due to the lack of access constraints in namespaces, access modifiers are not permitted.
- Only one accessibility, except private protected and protected internal, may be used by the user at once.
- Top-level types (those that are not nested in other types and can only have public or internal accessibility) have internal accessibility as their default setting.
- The default accessibility is applied depending on the context if no access modifier is supplied for a member declaration.
Conclusion
In conclusion, understanding C# access specifiers is vital for controlling data visibility and security. Mastering them empowers developers to create robust, efficient, and secure applications.
Take our free skill tests to evaluate your skill!

In less than 5 minutes, with our skill test, you can identify your knowledge gaps and strengths.