Arrays in C#: Create, Declare and Initialize the Arrays
Arrays in C#: An Overview
Explore the power of arrays in C# with our comprehensive guide. Learn how to declare, initialize, and manipulate arrays, unlocking the potential to store and process multiple elements efficiently. Whether you're a beginner or an experienced programmer, this article will enhance your understanding of C# arrays and their versatile applications.
What is an Array in C#?
In C#, an array is a data structure that allows you to store and manipulate a collection of elements of the same data type. Arrays provide a convenient way to work with groups of related variables, enabling efficient storage, retrieval, and iteration through the elements using index-based access.
The following figure illustrates an array representation.
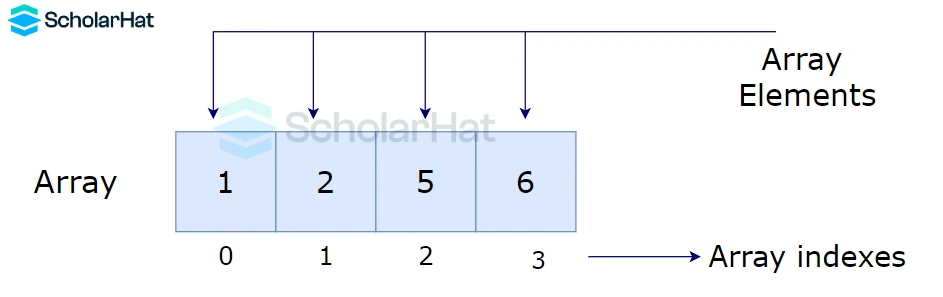
Array Declaration
Syntax
< Data Type > [ ] < Name_Array >
Explanation
- < Data Type >: It define the element type of the array.
- [ ]: It define the size of the array.
- < Name_Array >: It is the Name of array.
Example
int[] x; // can store int values
string[] s; // can store string values
double[] d; // can store double values
Student[] stud1; // can store instances of Student class which is custom class
Note: Only the Declaration of an array doesn’t allocate memory to the array. For that array must be initialized.
Read More - C# Interview Questions And Answers
Array Initialization
As said earlier, an array is a reference type so the new keyword is used to create an instance of the array. We can assign initialize individual array elements, with the help of the index.
Syntax
type [ ] < Name_Array > = new < datatype > [size];
Here, type specifies the type of data being allocated, size specifies the number of elements in the array, and Name_Array is the name of an array variable. And new will allocate memory to an array according to its size.
Examples: To Show Different ways for the Array Declaration and Initialization
Example 1 :
// defining array with size 5.
// But not assigns values
int[] intArray1 = new int[5];
The above statement declares & initializes int type array that can store five int values. The array size is specified in square brackets([]).
Example 2 :
// defining array with size 5 and assigning
// values at the same time
int[] intArray2 = new int[5]{1, 2, 3, 4, 5};
The above statement is the same as, but it assigns values to each index in {}.
Example 3:
// defining array with 5 elements which
// indicates the size of an array
int[] intArray3 = {1, 2, 3, 4, 5};
In the above statement, the value of the array is directly initialized without taking its size. So, array size will automatically be the number of values which is directly taken.
Initialization of an Array after Declaration
Arrays can be initialized after the declaration. It is not necessary to declare and initialize at the same time using the new keyword. However, Initializing an Array after the declaration, it must be initialized with the new keyword. It can’t be initialized by only assigning values.
Example:
// Declaration of the array string[] str1, str2;
// Initialization of array
str1 = new string[5]{ “Element 1”, “Element 2”, “Element 3”, “Element 4”, “Element 5” };
str2 = new string[5]{ “Element 1”, “Element 2”, “Element 3”, “Element 4”, “Element 5” };
Note: Initialization without giving size is not valid in C#. It will give a compile-time error.
Example: Wrong Declaration for initializing an array
// compile-time error: must give size of an array
int[] intArray = new int[]; // error : wrong initialization of an array
string[] str1;
str1 = {“Element 1”, “Element 2”, “Element 3”, “Element 4” };
Example
using System;
class Program
{
static void Main()
{
// Declare and initialize an integer array
int[] numbers = { 1, 2, 3, 4, 5 };
// Print array elements to the console
Console.WriteLine("Array Elements:");
for (int i = 0; i < numbers.Length; i++)
{
Console.WriteLine(numbers[i]);
}
}
}
Explanation:
In this code in the C# Compiler, an integer array named numbers is declared and initialized with values 1, 2, 3, 4, and 5. The program then iterates through the array and prints each element to the console. When you run this program, it will display the array elements as shown in the output comments.
Output:
Array Elements:
1
2
3
4
5
Accessing Array Elements
In C#, you access array elements using square brackets []. Specify the index inside the brackets to retrieve or modify the element. Indexing starts at 0, so array[0] accesses the first element. Ensure the index is within the array bounds to prevent out-of-range exceptions.
using System;
class Program
{
static void Main()
{
// Declare and initialize an array
int[] numbers = { 10, 20, 30, 40, 50 };
// Accessing array elements
Console.WriteLine("Array Elements:");
for (int i = 0; i < numbers.Length; i++)
{
Console.WriteLine("Element at index {0}: {1}", i, numbers[i]);
}
}
}
Explanation
This C# program initializes an integer array named "numbers" with five values. It then uses a for loop to iterate through the array, printing the index and corresponding element value to the console. This demonstrates how to declare, initialize, and access elements of an array in C#.
Output
Array Elements:Element at index 0: 10
Element at index 1: 20
Element at index 2: 30
Element at index 3: 40
Element at index 4: 50
Changing Array Elements
In C#, you can change array elements by accessing them using their index and assigning a new value. For example: myArray[index] = newValue; This will replace the element at the specified index with the new value.
using System;
class Program
{
static void Main()
{
// Creating and initializing an array
int[] numbers = { 1, 2, 3, 4, 5 };
// Displaying the original array
Console.WriteLine("Original Array:");
DisplayArray(numbers);
// Changing array elements
numbers[0] = 10;
numbers[2] = 30;
// Displaying the modified array
Console.WriteLine("\nModified Array:");
DisplayArray(numbers);
}
static void DisplayArray(int[] arr)
{
// Displaying elements of the array
for (int i = 0; i < arr.Length; i++)
{
Console.Write(arr[i] + " ");
}
Console.WriteLine();
}
}
Explanation
This C# program creates and initializes an integer array called "numbers." It then displays the original array using a custom method, modifies two elements of the array, and displays the modified array. Finally, it waits for user input before exiting. This demonstrates array creation, modification, and custom method usage in C#.
Output
Original Array:
1 2 3 4 5
Modified Array:
10 2 30 4 5
Finding the Length of an Array
In C#, you can find the length of an array using the Length property. For example, if you have an array called myArray, you can find its length using int length = myArray.Length;. This property returns the number of elements in the array.
using System;
class Program
{
static void Main()
{
int[] array = { 1, 2, 3, 4, 5 };
int length = array.Length;
Console.WriteLine("The length of the array is: " + length);
}
}
Explanation
This C# program in the C# Editor initializes an integer array "array" with five elements. It then calculates the length of the array using the "Length" property and prints the result, which is the number of elements in the array, to the console. In this case, it prints "The length of the array is: 5."
Output
The length of the array is: 5
Additional Array Operations
In addition to the basic operations covered so far, arrays in C# provide several other useful operations that you can perform. Here are a few examples:
Sorting an Array
You can sort the elements of an array in ascending or descending order using the Array.Sort() method. This method rearranges the elements of the array in place.
int[] numbers = { 3, 1, 4, 2, 5 };
Array.Sort(numbers);
Reversing an Array
You can reverse the order of the elements in an array using the Array.Reverse() method. This method reverses the elements of the array in place.
int[] numbers = { 1, 2, 3, 4, 5 };
Array.Reverse(numbers);
Searching for an Element
You can search for a specific element in an array using the Array.IndexOf() method. This method returns the index of the first occurrence of the specified element in the array.
int[] numbers = { 1, 2, 3, 4, 5 };
int index = Array.IndexOf(numbers, 3)
These are just a few examples of the operations you can perform on arrays in C#. Arrays provide a powerful and flexible way to store and manipulate data in your programs.
Conclusion
In this comprehensive guide, We have learned how to declare and initialize arrays, access their elements, change values, and determine their length. By applying the knowledge gained from this article, you will be well-equipped to leverage the power of arrays in your C# projects.
Take our free skill tests to evaluate your skill!

In less than 5 minutes, with our skill test, you can identify your knowledge gaps and strengths.