Method Parameters in C#: Types Explained with Examples
C# Method Parameters: An Overview
C# method parameters are essential components of function signatures, allowing developers to pass values into methods and return results. Understanding how to define and work with parameters is fundamental for effective C# programming.
Method Parameters in C#
In C#, method parameters are variables declared in a method's signature, defining the data that a method can accept. They enable passing values into methods for computation and allow methods to return results. Parameters specify the type and name of the data expected by the method, enhancing the flexibility and reusability of functions in C# programming.
Types of Method Parameters in C#
C# contains the following types of Method Parameters:
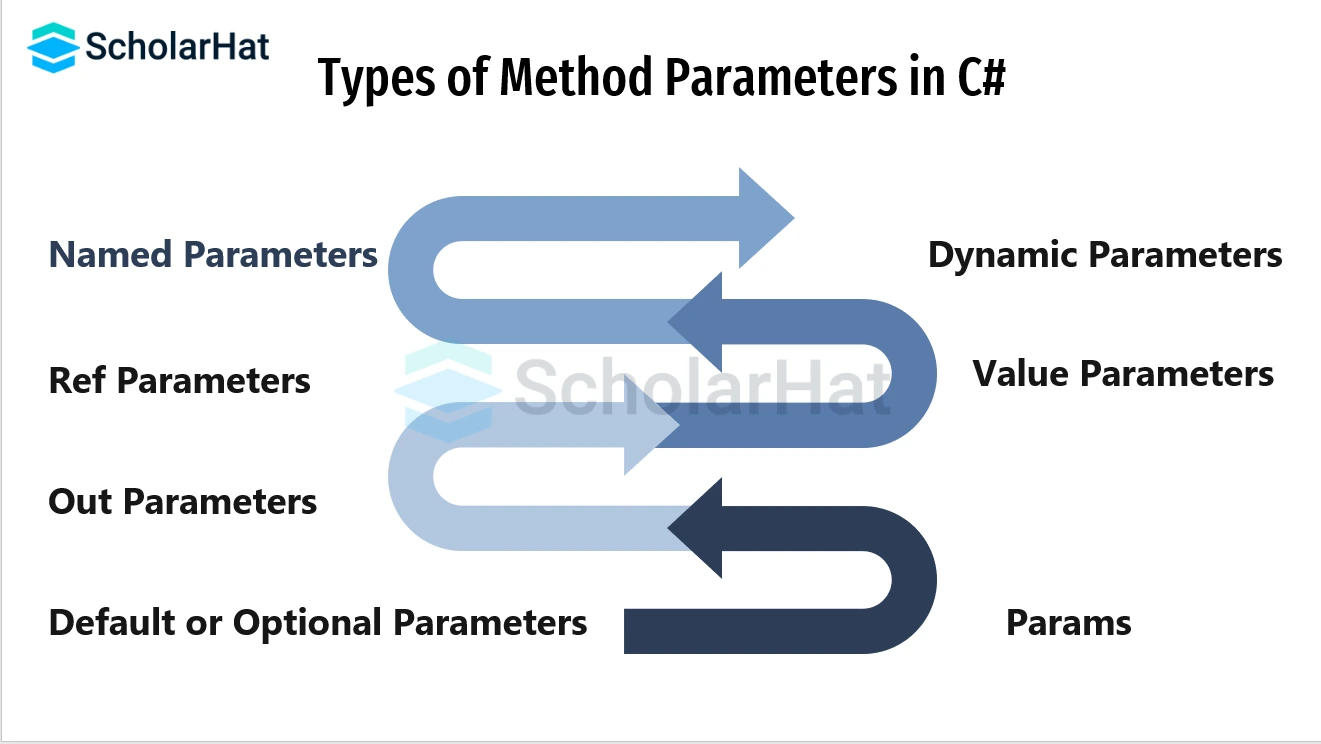
Named Parameters in C#
When using named parameters, you can specify the value of the parameter based on its name rather than the method's order. In other words, it gives us the option to forget about remembering parameters in the order they appeared. In C# 4.0, this concept is first introduced. When you use a method that has a greater number of parameters, your program becomes simpler to understand. But keep in mind that named parameters must always come after fixed arguments; if you try to provide a named parameter after a fixed argument, the compiler will throw an error.
Example
// C# program to illustrate the// concept of the named parameters
using System;
public class DNT {
// addstr contain three parameters
public static void addstr(string s1, string s2, string s3)
{
string result = s1 + s2 + s3;
Console.WriteLine("Final string is: " + result);
}
// Main Method
static public void Main()
{
// calling the static method with named
// parameters without any order
addstr(s1: "Dot", s2: "Net", s3: "Tricks");
}
}
Explanation
This C# program in the C# Compiler demonstrates the use of named parameters. The addstr method concatenates three strings and prints the result. When calling the method, named parameters (s1, s2, and s3) are used, allowing the values to be passed out of order for improved readability and clarity.
Output
Final string is: DotNetTricks
Read More - C Sharp Interview Questions
Ref Parameters in C#
In C#, the ref keyword is used to pass value types by reference. Or, we could state that when control returns to the calling method, any changes made to this argument in the method will be reflected in that variable. The ref parameter fails to satisfy the property. Prior to passing to the ref, the parameters in the ref parameters must be initialized. When the called method additionally needs to modify the value of the passed parameter, supplying a value through the ref parameter can be advantageous.
Example
// C# program to illustrate the concept of ref parameter
class DNT {
// Main Method
public static void Main()
{
// Assigning value
string val = "Dog";
// Pass as a reference parameter
CompareValue(ref val);
// Display the given value
Console.WriteLine(val);
}
static void CompareValue(ref string val1)
{
// Compare the value
if (val1 == "Dog")
{
Console.WriteLine("Matched!");
}
// Assigning new value
val1 = "Cat";
}
}
Explanation
This C# program demonstrates the use of a ref parameter. The method CompareValue takes a string parameter by reference. If the parameter is initially "Dog", it prints "Matched!" and then changes the parameter's value to "Cat". The modified value is then printed in the Main method, showing how ref parameters allow direct modification of the original variable.
Output
Matched!
Cat
Out Parameters in C#
In C#, the out keyword is used to deliver arguments to methods as a reference type. When a method returns several values, it is typically used. The out argument fails the test for the property. Initializing parameters before it flows to out is not essential. When a method returns numerous values, specifying parameters all throughout the method is helpful.
Example
// C# program to illustrate the concept of out parameter
using System;
class DNT {
// Main method
static public void Main()
{
// Creating variable
// without assigning value
int num;
// Pass variable num to the method
// using out keyword
AddNum(out num);
// Display the value of num
Console.WriteLine("The sum of"
+ " the value is: {0}",num);
}
// Method in which out parameter is passed
// and this method returns the value of
// the passed parameter
public static void AddNum(out int num)
{
num = 40;
num += num;
}
}
Explanation
This C# program demonstrates the use of an out parameter. The method AddNum takes an out parameter (num), assigns it a value (40), doubles it within the method, and returns the modified value. The Main method passes an uninitialized variable as out parameter, receives the modified value, and prints it, illustrating how out parameters can return values from methods.
Output
The sum of the value is: 80
Default or Optional Parameters in C#
Optional parameters are just what their name implies—they are not required parameters. Excluding arguments for some parameters is beneficial. Alternatively, we could say that not all of the method's parameters need to be passed. In C# 4.0, this idea is introduced. Here, the definition of each and every optional parameter includes a default value. The default value is used if we do not supply any arguments to the optional parameters. Always defined at the end of the parameter list are the optional parameters. Or, to put it another way, the optional parameter is the last parameter of the method, constructor, etc.
Example
// C# program to illustrate the concept of optional parameters
using System;
class DNT {
// This method contains two regular
// parameters, i.e. ename and eid
// And two optional parameters, i.e.
// bgrp and dept
static public void detail(string ename,
int eid,
string bgrp = "A+",
string dept = "Review-Team")
{
Console.WriteLine("Employee name: {0}", ename);
Console.WriteLine("Employee ID: {0}", eid);
Console.WriteLine("Blood Group: {0}", bgrp);
Console.WriteLine("Department: {0}", dept);
}
// Main Method
static public void Main()
{
// Calling the detail method
detail("XYZ", 123);
detail("ABC", 456, "B-");
detail("DEF", 789, "B+",
"Software Developer");
}
}
Explanation
This C# program in the C# Editor showcases the use of optional parameters. The method detail accepts two mandatory parameters (ename and eid) and two optional parameters (bgrp and dept). When calling the method, you can provide values only for the mandatory parameters, and the optional parameters will use their default values if not specified, demonstrating flexibility and simplifying method calls.
Output
Employee name: XYZ
Employee ID: 123
Blood Group: A+
Department: Review-Team
Employee name: ABC
Employee ID: 456
Blood Group: B-
Department: Review-Team
Employee name: DEF
Employee ID: 789
Blood Group: B+
Department: Software Developer
Dynamic Parameters in C#
A new class of parameters known as a dynamic parameter is introduced in C# 4.0. Here, the parameters are sent dynamically, which implies that the compiler determines the type of the dynamic type variable at runtime rather than at compile-time. A dynamic keyword is used to generate the dynamic type variable.
Example
// C# program to illustrate the concept of the dynamic parameters
using System;
class DNT {
// Method which contains dynamic parameter
public static void mulval(dynamic val)
{
val *= val;
Console.WriteLine(val);
}
// Main method
public static void Main()
{
// Calling mulval method
mulval(30);
}
}
Explanation
This C# program demonstrates the use of dynamic parameters. The method mulval accepts a dynamic parameter, allowing it to accept different data types without compile-time type checking. In this example, it takes an integer, squares it, and prints the result. The dynamic keyword enables flexibility in parameter types at runtime, aiding polymorphic behaviour.
Output
900
Value Parameters in C#
It is a typical value parameter or the passing of value types by value in a method. As a result, when variables are supplied as values, they hold data or values rather than references. The original value recorded as an argument will not be reflected if you make any modifications to the value type parameter.
Example
// C# program to illustrate value parameters;
using System;
namespace HelloScholarsApp;
public class DNT
{
// Main Method
static public void Main()
{
// The value of the parameter is already assigned
string str1 = "Scholar";
string str2 = "Hat";
string res = addstr(str1, str2);
Console.WriteLine(res);
}
public static string addstr(string s1, string s2)
{
return s1 + s2;
}
}
Explanation
This C# program demonstrates value parameters. It defines a method addstr that takes two string parameters, concatenates them, and returns the result. In the Main method, two strings are passed to addstr, and the concatenated string is printed. Value parameters pass the values of the variables, ensuring the original data remains unchanged.
Output
ScholarHat
Params in C#
When the programmer is unsure of the number of parameters to be utilized, it can be helpful. You can pass any variable number of parameters by using params. There can be only one params keyword in a function declaration, and any additional parameters must come before the params keyword. If no arguments are given, the length of params will be 0.
Example
// C# program to illustrate params
using System;
namespace Examples {
class Scholars {
// function containing params parameters
public static int mulval(params int[] num)
{
int res = 1;
// foreach loop
foreach(int j in num)
{
res *= j;
}
return res;
}
static void Main(string[] args)
{
// Calling mulval method
int x = mulval(20, 49, 56, 69, 78);
// show result
Console.WriteLine(x);
}
}
}
Explanation
This C# program in the C# Online Compiler showcases the use of the params keyword. The mulval method accepts a variable number of integer parameters. It multiplies all the values passed and returns the result. In the Main method, mulval is called with multiple integers, demonstrating the flexibility of params to handle varying numbers of arguments. The output is the multiplication result of the provided integers.
Output
295364160
Conclusion
In conclusion, understanding C# method parameters is fundamental for effective programming. By mastering the intricacies of parameter types, passing mechanisms, and default values, developers can harness the full power of C# to create robust and flexible applications.
Take our free skill tests to evaluate your skill!

In less than 5 minutes, with our skill test, you can identify your knowledge gaps and strengths.